NodeJs introduction: toolkit overview
NodeJs introduction: toolkit overview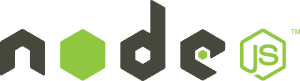
This article describes the basics of NodeJs: the shell, the package management and the structure of package.json; For more information about Node, click here.
Shell, read-eval-print-loop
Node provides an interactive shell, known as the Read-Eval-Print-Loop(REPL). The REPL reads input from the user, evaluates the input as Javascript code. It’s useful for debugging and for experimenting with small snippets of Javascript code. To launch node simply type node
on terminal.
REPL command
.help |
The command displays all of the available REPL commands |
.exit |
The command termiates the REPL |
.break |
The .break command is used to bail out of a multiline expression. |
.save <path_file> |
The .save command saves the current REPL session. You need to specify the complete path of file. |
.load <path_file> |
The .load command loads the current REPL session. You need to specify the complete path of file. |
.clear |
The .clear command has the same functionality of .break, it can be also used to reset the context. |
Package management system
The Node true strengths is its developer community and myriad of third-party modules. Keeping track of all of these modules is Node’s package manager, NPM.
NPM manages Node modules and their dependencies.
Installing packages
In order to use a module, you must install it on your machine. To install a package, type npm install
followed by package name, for example, to install the latest version of express, type:
npm install express
Node modules follow a major.minor.patch versioning scheme, the @ character is used to separate the package name from the version.
npm install express@1.0.0
If you want to install the latest patch of a certain release you must use the x
wildcard:
npm install express@1.0.X
You can also select version using relational version range descriptors: it select the most recent version that matches a given set of criteria. The relational version range descriptrs supported by NPM are listed in the following image:
Installing from URLs
In addition, npm allows packages to be installed directly from git
URLs:
npm install https://github.com/user/project
Updating packages
To determine if your packages are out of date, run: npm outdated
in your project directory. To update, outdated packages use npm update
.
Package locations
When packages are installed, they are saved on your local machine, the location is usually a subdirectory named node_modules
.
To determine package location, use the command:
npm root
To list all packages, use the command:
npm ls
└─┬ express@4.13.3
├─┬ accepts@1.2.13
│ ├─┬ mime-types@2.1.8
│ │ └── mime-db@1.20.0
│ └── negotiator@0.5.3
├── array-flatten@1.1.1
├── content-disposition@0.5.0
...
Global packages
Local packages must be installed in every project using them.
The global packages needs to be installed in one location, They normally contain command line tools, which should be included in the PATH environment variable.
To install packages globally, run:
npm install -g
Youn can process global packages by adding the -g
(or --global
) option to most npm
commands.
Package.json
NPM understands the concept of module dependencies using the package.json, it stores all relevant information about the package, it must be located in your project’s root directory. The following code specifies minimal informations witten in simple package.json file:
Main entry point
Node needs some way of identifying its main entry point. The main
field it’s used to specify the entry point:
Dependencies
The dependencies
field defines dependencies of current packages. It map package names to version strings:
Developmental dependencies
Many packages have dependencies that are used only for testing and development, devDependencies
field specifies all dependencies used ONLY in the Development environment:
Scripts definition
The scripts
field, contains a mapping of npm commangs to script commands. The script commands, which can be any executable commands, are run in an external shell process:
To execute commands you need to type npm "command_name"
in shell.
There are also commands that are executed when a certain events occur. For example, the install
and unistall
.
For a complete listing of available script commands, run npm help scripts.
Other fields
A number of other fields are commonly found in the package.json
, you can run npm help json
to see more configuration fields.
require() function
require()
function is used to import modules into your project.
require()
accepts a single argument, a string specifying the module to load. If the specified module path exists, require()
returns an object that can be used to interface with the module:
var express= require("express");
Module types
There are two different types of modules:
- Core modules: core modules are modules compiled into the Node binary. The core modules are located in the
lib
of the Node source code; - File modules: file modules are non-core modules loaded from the system. They can be specified using absolute paths or relative paths;
Resolving a module location
If you are interested about an module path you can use require.resolve("module_name");
to obtain the module path.